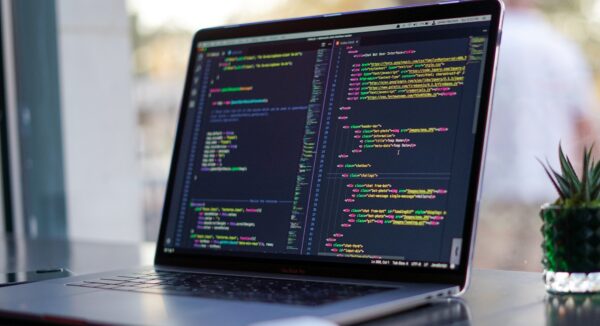
- Software Development
Testing code is a fundamental part of every developer’s job, followed by QA analysts ensuring the software’s quality. Fortunately, modern technologies like Cypress make this process faster and more efficient.
This modern end-to-end testing framework simplifies the creation of automated tests for web applications. Unlike other tools, Cypress is designed specifically for modern web environments, providing a faster, more reliable, and user-friendly testing experience.
Explore the key advantages of Cypress and how it can enhance your software testing workflow.
Cypress is a testing tool designed to streamline the process of writing, running, and debugging tests. It enables developers to write tests in JavaScript, leveraging an intuitive API that facilitates software development.
Additionally, it offers an interactive interface that enables real-time test visualization, enhancing the overall testing process. This feature enables QA analysts to track test scripts as they run, facilitating the identification of areas requiring adjustments.
Choosing the appropriate tool for automated testing is essential for enhancing speed and operational efficiency. Cypress is a leading option for several reasons:
It also boasts a strong community and comprehensive documentation. It provides extensive resources and support for developers and QA professionals.
Cypress utilizes JavaScript for test development, ensuring seamless compatibility with contemporary web applications. Given the prevalence of JavaScript in the development of front-end applications, Cypress enables developers to utilize the same language for both development and testing, thereby streamlining the workflow.
The primary distinction between Cypress and Selenium lies in their architecture:
While Selenium boasts greater flexibility in terms of browser and language compatibility (Java, C#, Python, Ruby), Cypress excels in speed, ease of use, and debugging efficiency with its JavaScript-based API and real-time feedback.
Cypress facilitates a range of software tests to ensure seamless functionality, including:
Integration testing checks that the different parts of the application work together. For example, Cypress can be used to test communication between the database layer and the user interface layer.
Cypress is used to test the user interface and ensure that users can interact with the application without navigation problems.
Cypress is useful for testing application performance and identifying bottlenecks that can affect the user experience, such as loading speed.
Try using Cypress to check that planned new features won’t negatively impact existing features.
You can also test the application to ensure that it complies with web accessibility guidelines.
Cypress also helps identify vulnerabilities that can be exploited by hackers.
Cypress seamlessly integrates with several technologies and frameworks, including:
By integrating Cypress within a CI/CD pipeline, test execution can be automated on the occurrence of code changes, thereby ensuring continuous quality assurance.
Want to see how Cypress works in practice? Check out our step-by-step installation and configuration guide!
To install Cypress in your development environment using npm (Node.js package manager), run the following command:
Now you need to configure it for use in your automated tests. To do this, you need to create a configuration file in your project, and simply run the following command in your terminal.
This will cause Cypress to open in your browser and create the cypress.json file in the project – this file is responsible for storing the Cypress settings.
Next you need to define the URL of your web application in baseUrl within the cypress.json file. For example:
This defines the base URL for your web application at http://localhost:3000.
To maintain an efficient testing workflow, follow these best practices:
Using environment variables is a good way to keep your credentials and sensitive information out of the source code. Use environment variables to store information such as URLs, login credentials and other sensitive information.
You may have noticed that the most important thing is to keep your files organised in a way that makes sense to your development team and makes it easier to maintain tests over time.
To use Cypress correctly, there are a few aspects of its structure you need to remember, such as:
In the example below we have the following folders and additional files:
Learn about some of the main Cypress commands that will make the framework easier to use:
To find elements on screen:
To interact with screen elements:
Assertions for our tests:
Let’s look at two test cases: the first checks whether an error message is displayed if the user’s credentials are incorrect. The second checks whether the user is redirected to the dashboard if the credentials are correct.
To do this, before each test we use the cy.visit() command to visit the login page. Next, we use cy.get() and .type() to fill in the email and password fields, and cy.get() and .click() to click on the login button. Finally, the cy.url() command is responsible for checking that the user is redirected to the correct page after logging in. It’s worth noting that the tests can be adapted to the specific needs of your application.
You can use a JSON file to store CSS selectors, which is particularly useful for large projects where there are many locators to manage, as it allows for easier organisation and maintenance.
Below is an example of how to create a JSON file with locators and use it with Cypress:
In the example above, we have a locators.json file that contains the locators organised by page. To use these locators in the test, simply import the JSON file and use the cy.get(nameOfLocator) syntax to locate the element on the page.
Using a JSON file to store the locators simplifies code maintenance by allowing the locators to be updated centrally in a single file.
Below is an example where we use the cypress-axios plugin to make HTTP requests to the API. We also use the cypress-aliases plugin to create an alias to the base API URL that can be referenced by @api in the tests.
In the first test, we’ll check that the API responds correctly by making a GET request to the API’s base URL.
In the second test, we’ll check that the API returns the expected response. We’ll do this by making a GET request to the API’s /hello route.
And the third test is to check that the API returns valid XML by making a GET request to the API’s /xml route and validating the XML returned.
It’s important to remember that back-end testing with Cypress is more limited than front-end testing because there is no user interaction with the application.
However, it is still possible to check the API response and validate the expected behaviour of the application. See the figure below:
Here’s an example of a request file:
See an example of a payload file:
Below is an example of a functional test in Cypress. In the first block, we perform the interactions of a registration; in the second, we click on the registration button which calls the post method; in the last, the test validations.
Cypress is a powerful end-to-end testing tool with an intuitive JavaScript API, easy installation, interactive debugging and strong community support. Its fast execution and integrated browser experience make it an excellent choice for modern web applications.
Looking for expert guidance? Meet our nearshore development teams and improve your software testing strategy and effectively mitigate risk.
Here are the answers to the main questions about Cypress:
Cypress is an end-to-end testing framework designed for web applications, offering fast execution and real-time debugging.
Cypress is easy to set up, has an intuitive interface, executes tests quickly, and simplifies debugging, making it ideal for web testing.
Cypress is written in JavaScript and is widely compatible with modern web applications.